Amplitude
Amplitude is a powerful mobile analytics service. With Adapty, you can easily send events to Amplitude, see how users behave, and then make smart decisions.
Adapty provides a complete set of data that lets you track subscription events from stores in one place and sends it to your Amplitude account. This allows you to match your user behavior with their payment history in Amplitude, and inform your product decisions.
How to set up Amplitude integration
Within Adapty, you can set up separate flows for production and test events from the Apple or Stripe sandbox environment or Google test account.
- For production events, enter the Production API keys from the Amplitude dashboard, with a unique API key for each platform: iOS, Android, and Stripe.
- For test events, use the Sandbox fields as needed.
To set up the Amplitude integration:
- Open Integrations -> Amplitude in your Adapty Dashboard.
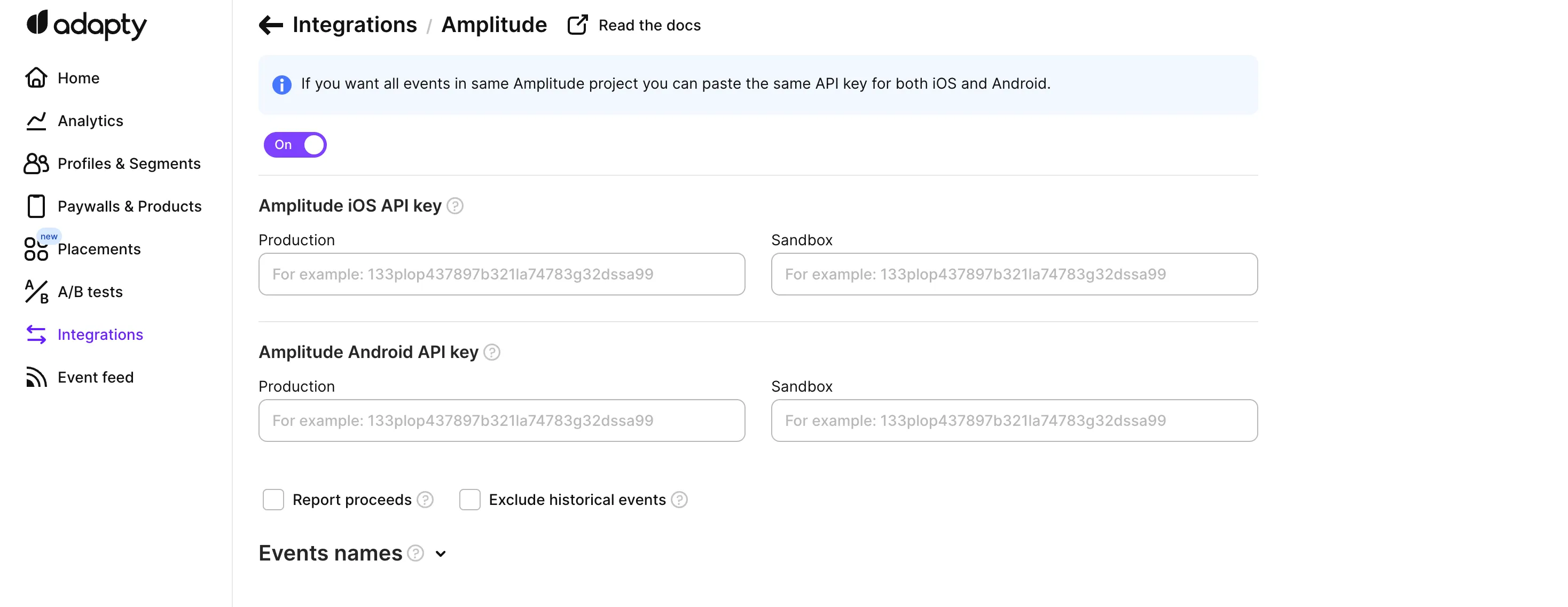
-
Toggle on Amplitude integration to enable it.
-
Fill in the integration fields:
Field Description Amplitude iOS/ Android/ Stripe API key Enter the Amplitude API Key for iOS/ Android/ Stripe into Adapty. Locate it under Project settings in Amplitude. For help, check Amplitude docs. Start with Sandbox keys for testing, then switch to Production keys after successful tests. -
Optional settings for further customization:
Parameter Description How the revenue data should be sent Choose whether to send gross revenue or revenue after taxes and commissions. See Store commission and taxes for details. Exclude historical events Choose to exclude events before Adapty SDK installation, preventing duplicate data. For example, if a user subscribed on January 10th but installed the Adapty SDK on March 6th, Adapty will only send events from March 6th onward. Send User Attributes Select this option to send user-specific attributes like language preferences. Always populate user_id Adapty automatically sends device_id
asamplitudeDeviceId
. Foruser_id
, this setting defines behavior:- ON: Sends Adapty
profile_id
ifamplitudeUserId
orcustomer_user_id
aren’t available. - OFF: Leaves
user_id
empty if neither ID is available.
- ON: Sends Adapty
-
Choose the events you want to receive and map their names.
-
Click Save to confirm your changes.
Once you click Save, Adapty will start sending events to Amplitude.
In addition to events, Adapty sends subscription status and the subscription product ID to Amplitude user properties.
Events and tags
Below the credentials, there are three groups of events you can send to Amplitude from Adapty. Simply turn on the ones you need. Check the full list of the events offered by Adapty here.
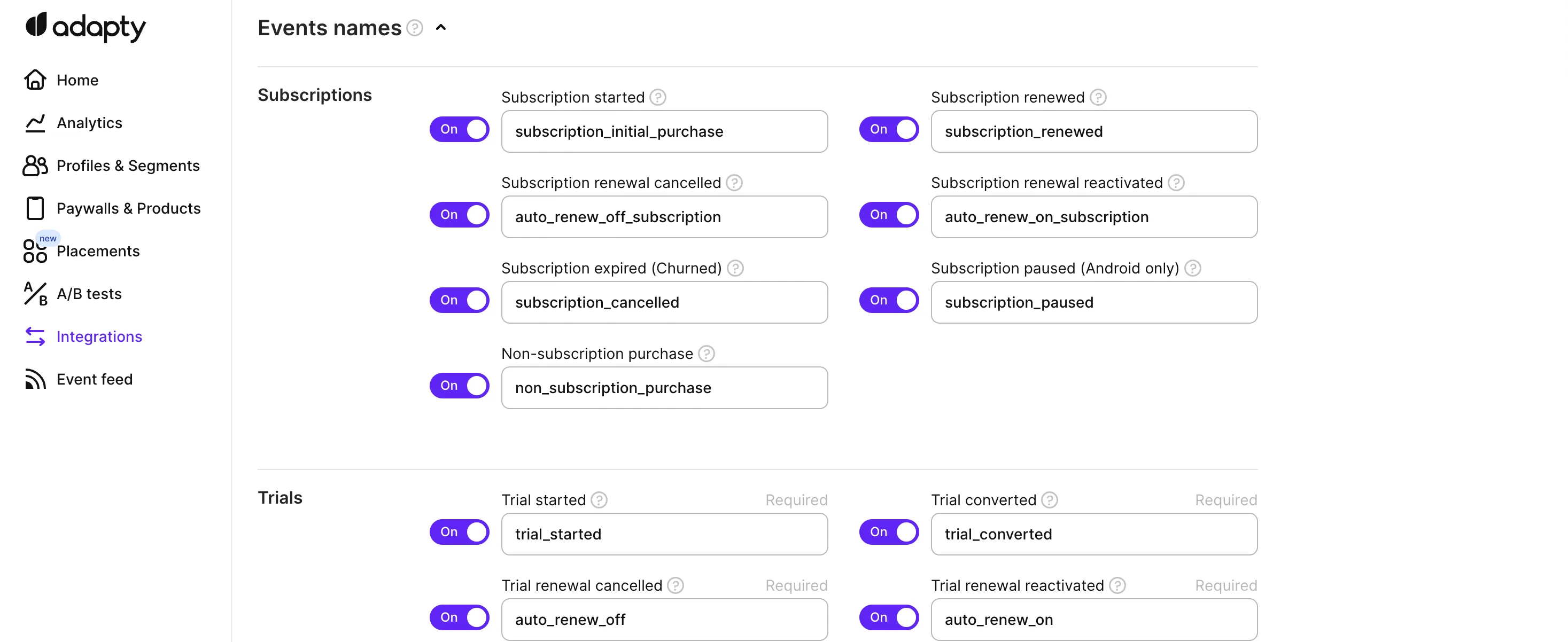
We recommend using the default event names provided by Adapty. But you can change the event names based on your needs. Adapty will send subscription events to Amplitude using a server-to-server integration, allowing you to view all subscription events in your Amplitude dashboard.
SDK configuration
Use the setIntegrationIdentifier()
method to set either amplitudeDeviceId
or amplitudeUserId
. If neither is set, Adapty will default to using your user ID (customerUserId
). If customerUserId
is also absent, Adapty will handle user_id
based on the Always populate user_id checkbox:
- If selected, Adapty will set
user_id
to the Adaptyprofile_id
. - If cleared,
user_id
will not be set.
Make sure that any user ID you use to send data to Amplitude from your app matches the one you send to Adapty.
- iOS (Swift)
- Android (Kotlin)
- Flutter (Dart)
- Unity (C#)
- React Native (TS)
import Amplitude
do {
try await Adapty.setIntegrationIdentifier(
key: "amplitude_user_id",
value: Amplitude.instance().userId
)
try await Adapty.setIntegrationIdentifier(
key: "amplitude_device_id",
value: Amplitude.instance().deviceId
)
} catch {
// handle the error
}
//for Amplitude maintenance SDK (obsolete)
val amplitude = Amplitude.getInstance()
val amplitudeDeviceId = amplitude.getDeviceId()
val amplitudeUserId = amplitude.getUserId()
//for actual Amplitude Kotlin SDK
val amplitude = Amplitude(
Configuration(
apiKey = AMPLITUDE_API_KEY,
context = applicationContext
)
)
val amplitudeDeviceId = amplitude.store.deviceId
val amplitudeUserId = amplitude.store.userId
//
Adapty.setIntegrationIdentifier("amplitude_user_id", amplitudeUserId) { error ->
if (error != null) {
// handle the error
}
}
Adapty.setIntegrationIdentifier("amplitude_device_id", amplitudeDeviceId) { error ->
if (error != null) {
// handle the error
}
}
import 'package:amplitude_flutter/amplitude.dart';
final Amplitude amplitude = Amplitude.getInstance(instanceName: "YOUR_INSTANCE_NAME");
try {
await Adapty().setIntegrationIdentifier(
key: "amplitude_user_id",
value: amplitude.getUserId(),
);
await Adapty().setIntegrationIdentifier(
key: "amplitude_device_id",
value: amplitude.getDeviceId(),
);
} on AdaptyError catch (adaptyError) {
// handle the error
} catch (e) {
// handle the error
}
using AdaptySDK;
Adapty.SetIntegrationIdentifier(
"amplitude_user_id",
"YOUR_AMPLITUDE_USER_ID",
(error) => {
// handle the error
});
Adapty.SetIntegrationIdentifier(
"amplitude_device_id",
amplitude.getDeviceId(),
(error) => {
// handle the error
});
import { adapty } from 'react-native-adapty';
try {
await adapty.setIntegrationIdentifier("amplitude_device_id", deviceId);
await adapty.setIntegrationIdentifier("amplitude_user_id", userId);
} catch (error) {
// handle `AdaptyError`
}