How to add in-app subscriptions to an iOS app
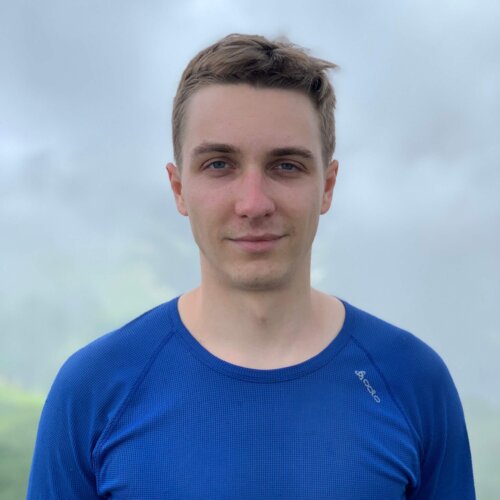
Updated: December 20, 2023
8 min read
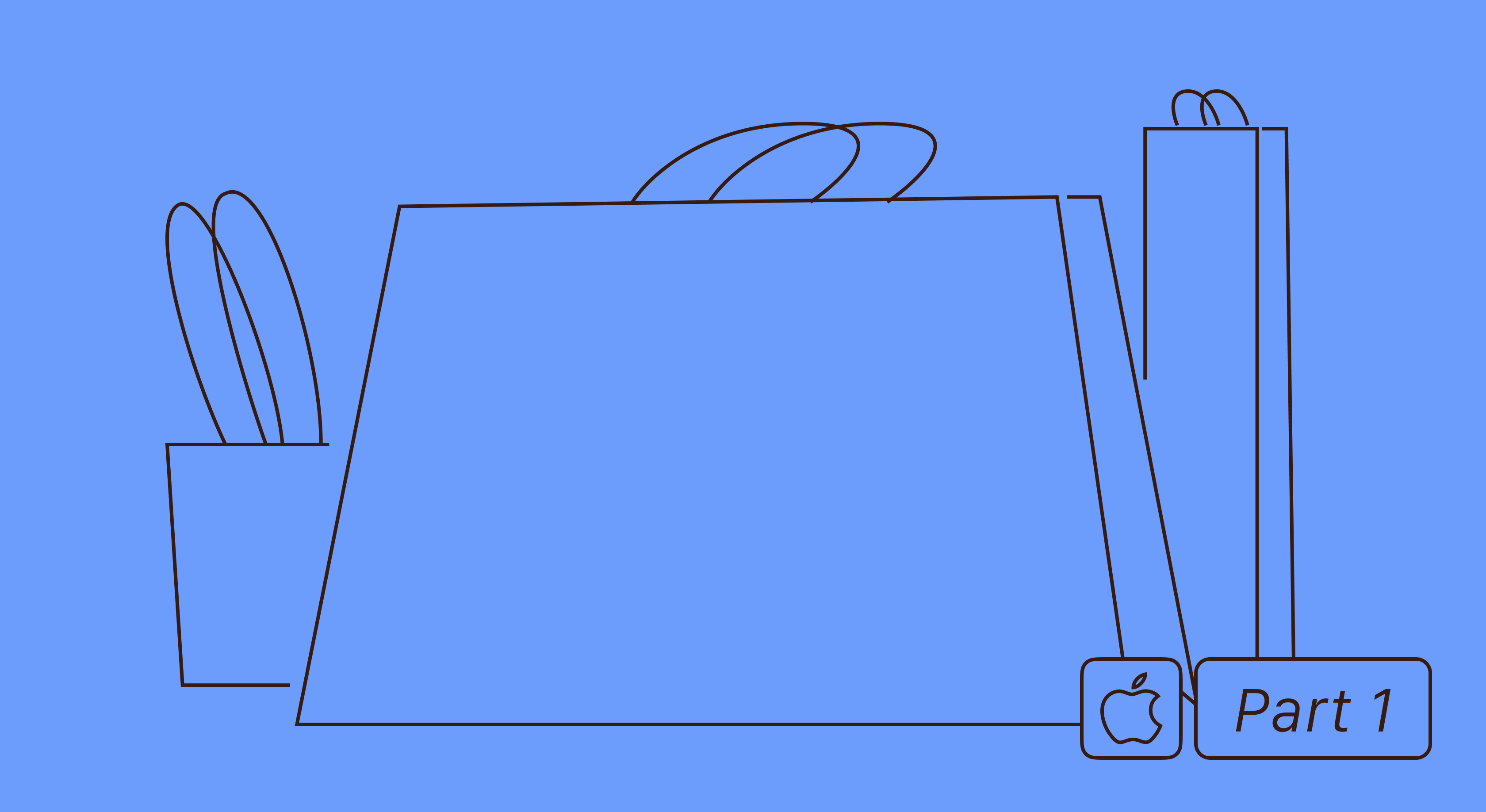
Subscriptions are one of the ways to monetize an app. Developers can get a recurrent income and provide users with up to date content. Unlike regular purchases, where Apple takes a 30% commission, this commission reduces to 15% in case the user is subscribed during one year or longer. Important: if the user cancels the subscription, this counter resets in 60 days.
With this article, we start our series of tutorials on integrating in-app purchases into an app. Here is what we’ll be talking about:
- iOS in-app purchases, part 1: configuration and adding to the project.
- iOS in-app purchases, part 2: initialization and purchases processing.
- iOS in-app purchases, part 3: testing purchases in XCode.
- iOS in-app purchases, part 4: server-side receipt validation.
- iOS in-app purchases, part 5: the list of SKError codes and how to handle them.
In this part we will learn how to:
- Create purchases in App Store Connect.
- Configure subscriptions: set a duration, price, trial periods.
- Get a list of purchases in-app.
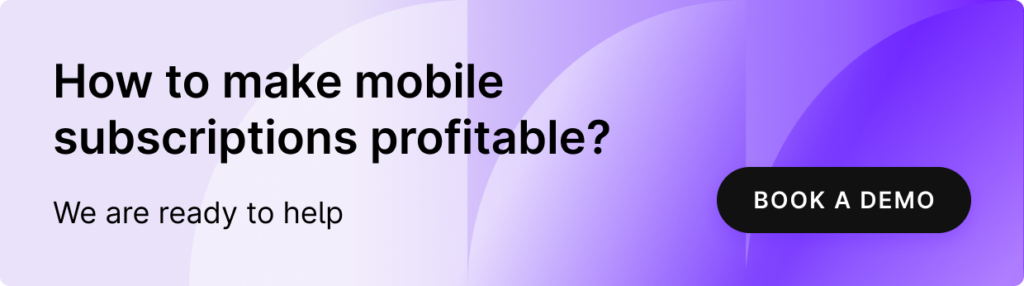
Creating iOS in-app purchases in App Store Connect
Before implementing your in-app purchases you have to:
- Pay for the Apple Developer account as a natural person or an entity.
- Accept all the agreements in App Store Connect. Updated agreements will appear in your App Store Connect account, it’s easy to notice them.
Let’s assume that our app will have two types of subscriptions: monthly and annual. Each of them will have a 7-day free trial.
First of all, we need to create a subscription group.
A Subscription Group is a set of subscriptions with the essential feature: the user cannot activate two subscriptions at the same time from the same group. Besides, all the introductory offers, such as trial subscriptions, are applied directly to the entire group. Groups serve to separate business logic in the application.
On the application page in App Store Connect, open the Subscriptions tab. Here, you will be able to create a Subscription Group by clicking the plus button or by clicking Create if the list is empty.
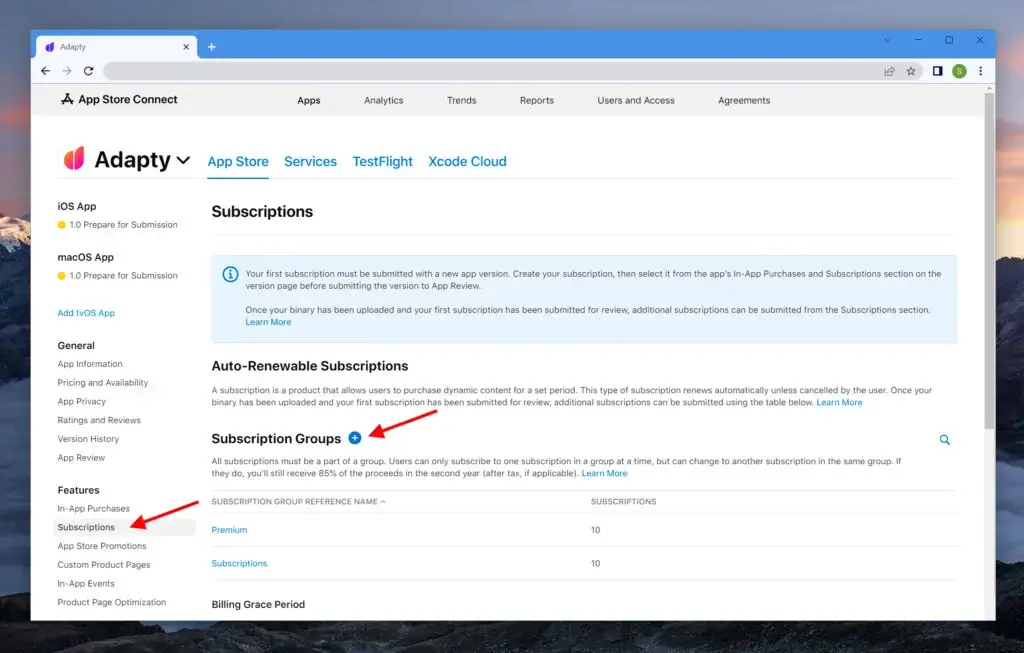
Let’s name the group Premium Access.
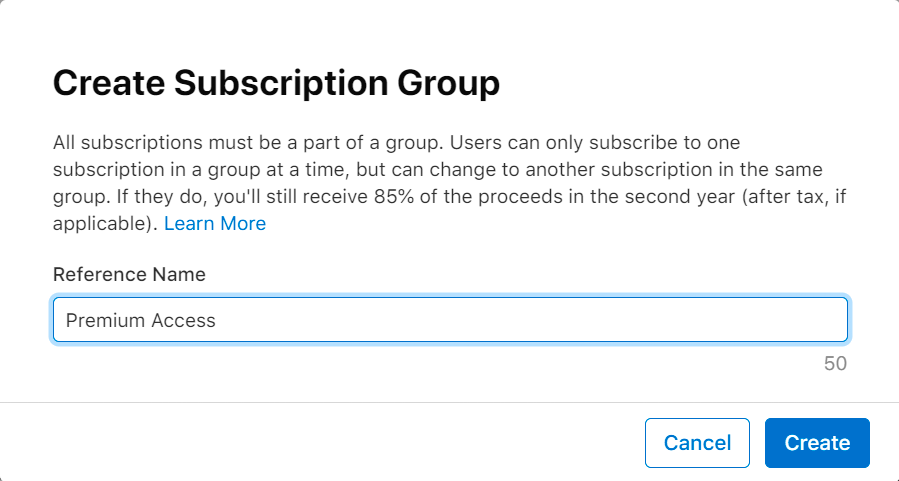
Once the subscription group is ready, let’s add the subscriptions by hitting Create.
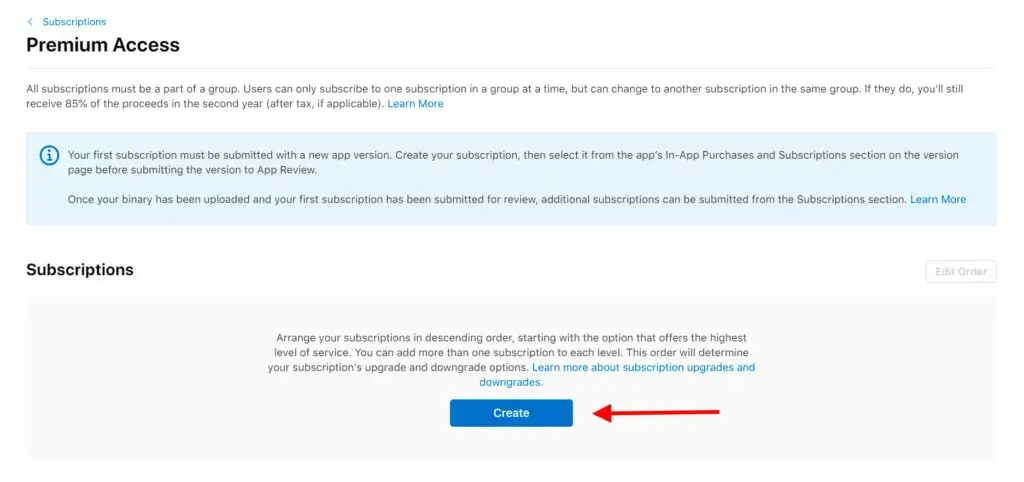
Now, let’s fill out the details:
- Reference Name is the title of your subscription in App Store Connect, as well as in Sales section and the reports
- Product ID is a unique product identifier, which is used in the application code
I would recommend choosing a simple Product ID name, which could give you as much information as possible. It’s better to indicate subscription duration to ease further analytics evaluation.
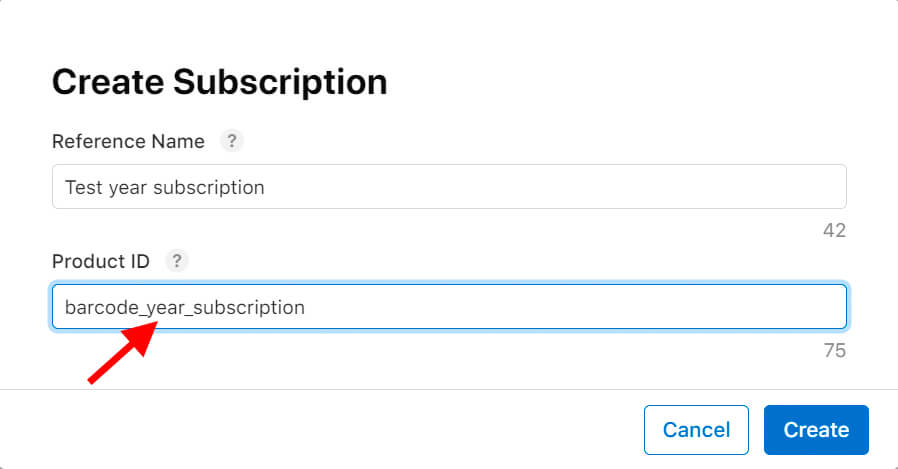
Add the second product following the previous instruction.
In the end, the interface of the subscriptions section will look like this:

Adding Subscription configuration for App Store
We added the purchases, but they are not ready yet to be used: status bar indicates Missing Metadata. It means we still haven’t added the information about pricing and subscription duration. We’ll fix it now.
Configuring subscription duration and pricing
Click on the product to configure it. Here we need to choose a Subscription Duration. In our case, we choose 1 Month or 1 Year, depending on the product.
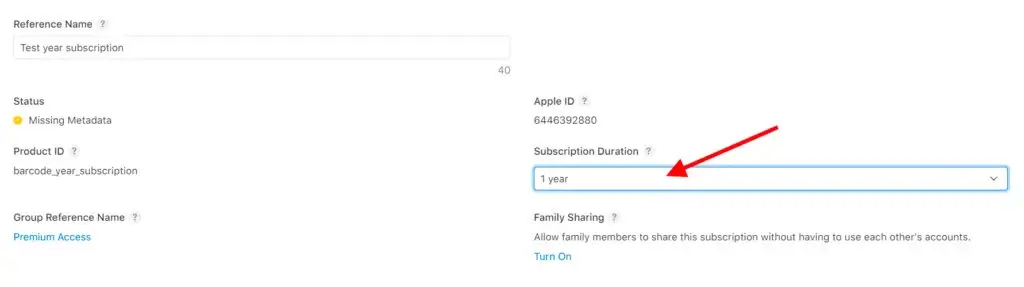
Then go lower to the Subscription Prices menu and click Add Subscription Price.
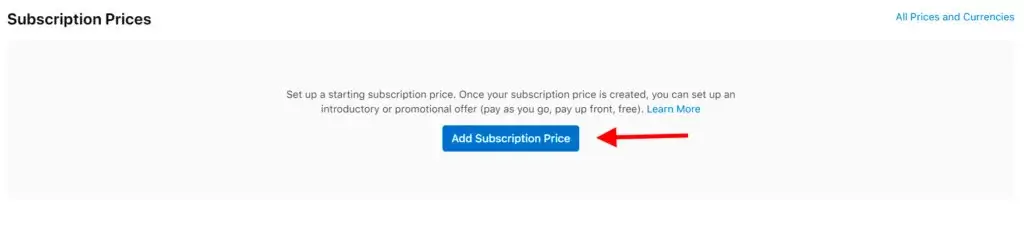
You can flexibly set prices depending on the country, but we will limit ourselves to automatic prices, in USD.
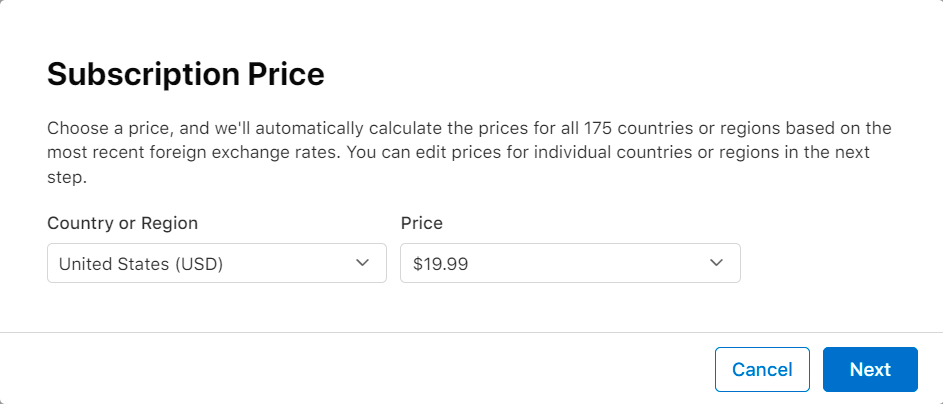
App Store Connect will automatically convert prices into another currency, it’s not clear how it works though. Probably you would want to change the price manually adjusting to your target market. When the duration and the prices are set, hit the Save button on the subscription page.
Activating free trial
One of the most popular ways to increase subscriptions is a free trial:
The user activates it and uses an application for free. If he doesn’t cancel the subscription, after the trial expires, the user is being charged automatically.
The developers love free trials because they work well. Let’s learn how to enable it.
Click (+) next to the title and choose Create Introductory Offer from the list:
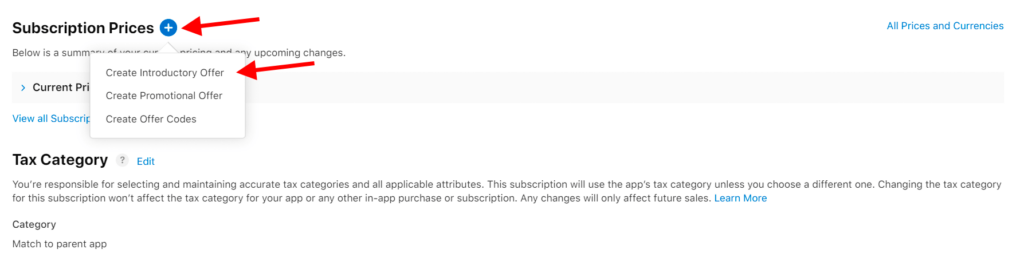
Set the countries and regions you want it to be enabled for.
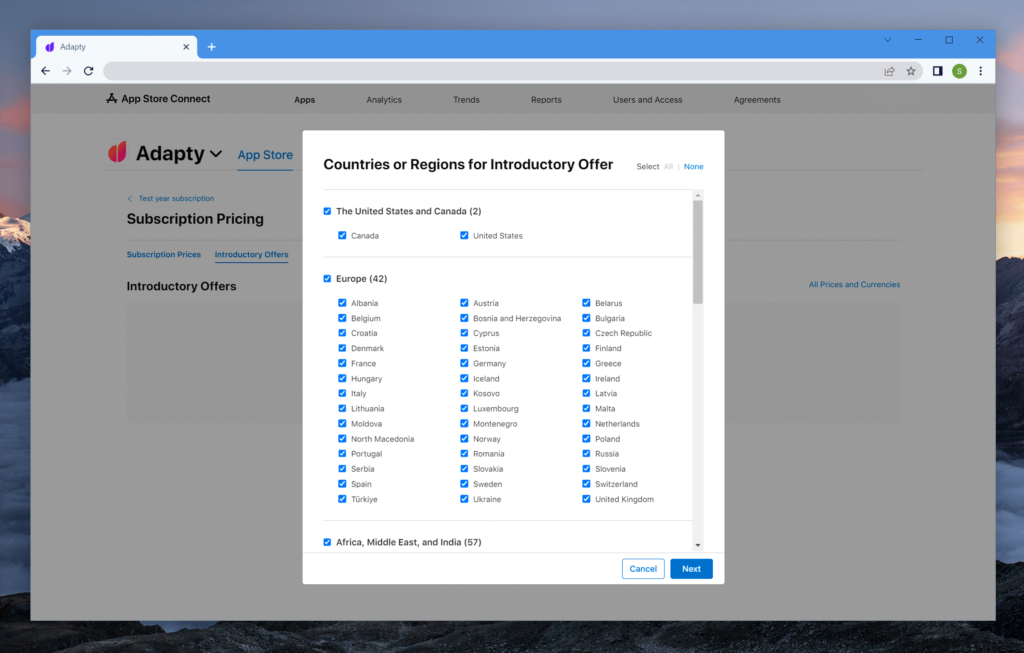
Choose the offer duration. You can set No End Date if you don’t want to limit yourself.
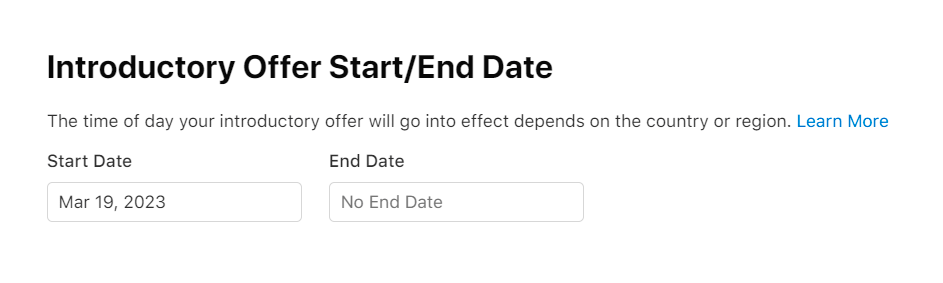
In the last step, you need to select an Offer Type.
As you can see in the next screenshot below, there are three types:
Pay as you go — a reduced-price use: the user pays a reduced price during the initial period and then becomes a regular subscriber with standard prices.
Pay up front — a prepay use: the user pays a fixed amount of money at once and gets an opportunity to use the app during the determined period, and then also becomes a regular subscriber.
Free — a free trial, after its expiry theuser may become a subscriber.
We choose the third one and set the duration for one week.
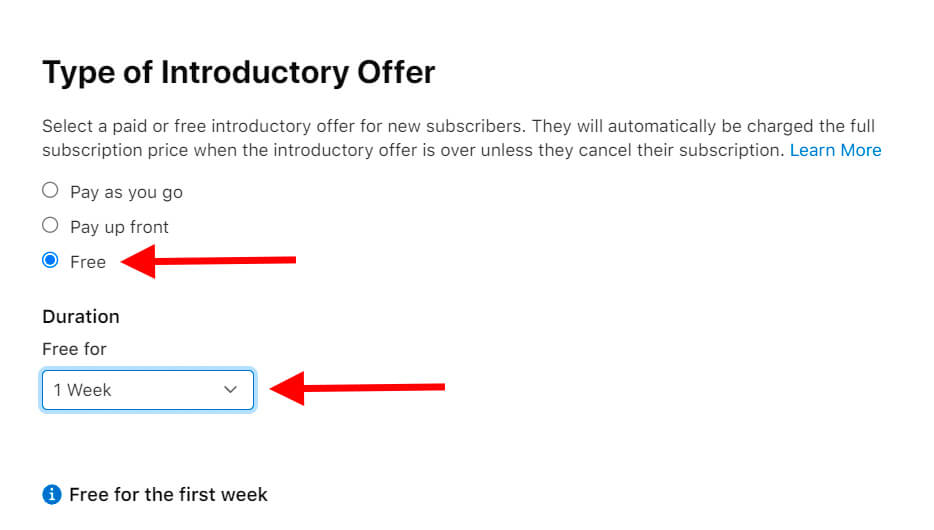
Save the settings and go to the next step.
Getting the list of SKProduct
Let’s check if the mobile app sees the purchases and then lay the groundwork for further realization of purchases.
It’s a good rule to create a class singleton for working with StoreKit. Such a class has only one instance in the entire app. A lot of productIdentifiers will store our purchases identifiers:
import StoreKit
class Purchases: NSObject {
static let `default` = Purchases()
private let productIdentifiers = Set(
arrayLiteral: "barcode_month_subscription", "barcode_year_subscription"
)
private var productRequest: SKProductsRequest?
func initialize() {
requestProducts()
}
private func requestProducts() {
// Will implement later
}
}
Only identifiers are not enough.
To fully use the purchases you need to get: price, currency, localization, discounts.
SKProduct class returns all this information and even more. To get this information, we need to request Apple. Create an object SKProductsRequest, assign “delegate” (there you will receive the result). Call the method start(), which initialize an asynchronous procedure:
private func requestProducts() {
productRequest?.cancel()
let productRequest = SKProductsRequest(productIdentifiers: productIdentifiers)
productRequest.delegate = self
productRequest.start()
self.productRequest = productRequest
}
If the operation is successful, productsRequest(didReceive response:) method will be called, which will contain all the relevant information:
extension Purchases: SKProductsRequestDelegate {
func productsRequest(_ request: SKProductsRequest, didReceive response: SKProductsResponse) {
guard !response.products.isEmpty else {
print("Found 0 products")
return
}
for product in response.products {
print("Found product: (product.productIdentifier)")
}
}
func request(_ request: SKRequest, didFailWithError error: Error) {
print("Failed to load products with error:n (error)")
}
}
If everything is successful, there will be two lines in the log:
Found product: barcode_monthly_subscription
Found product: barcode_year_subscription
However, if there is an error, then SKProduct with such an ID will not be returned. It may happen if the product has become invalid due to any reason.
That’s it.
The next article will be focused on how to make in-app purchases: open/close transactions, handle errors, validate receipt, and more.
If you liked it, give us ⭐ on GitHub
Good to read:
Apple’s Fiscal Calendar 2023 ⟶Recommended posts