Getting started with SwiftUI framework
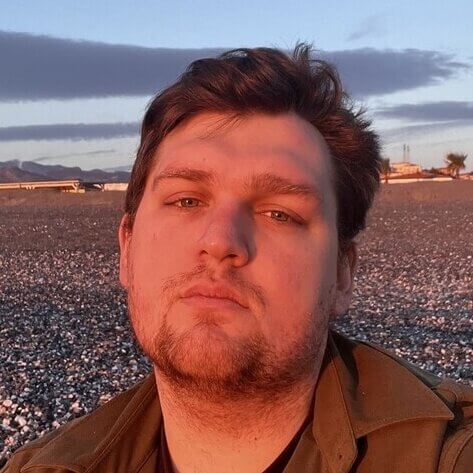
Updated: December 22, 2023
16 min read
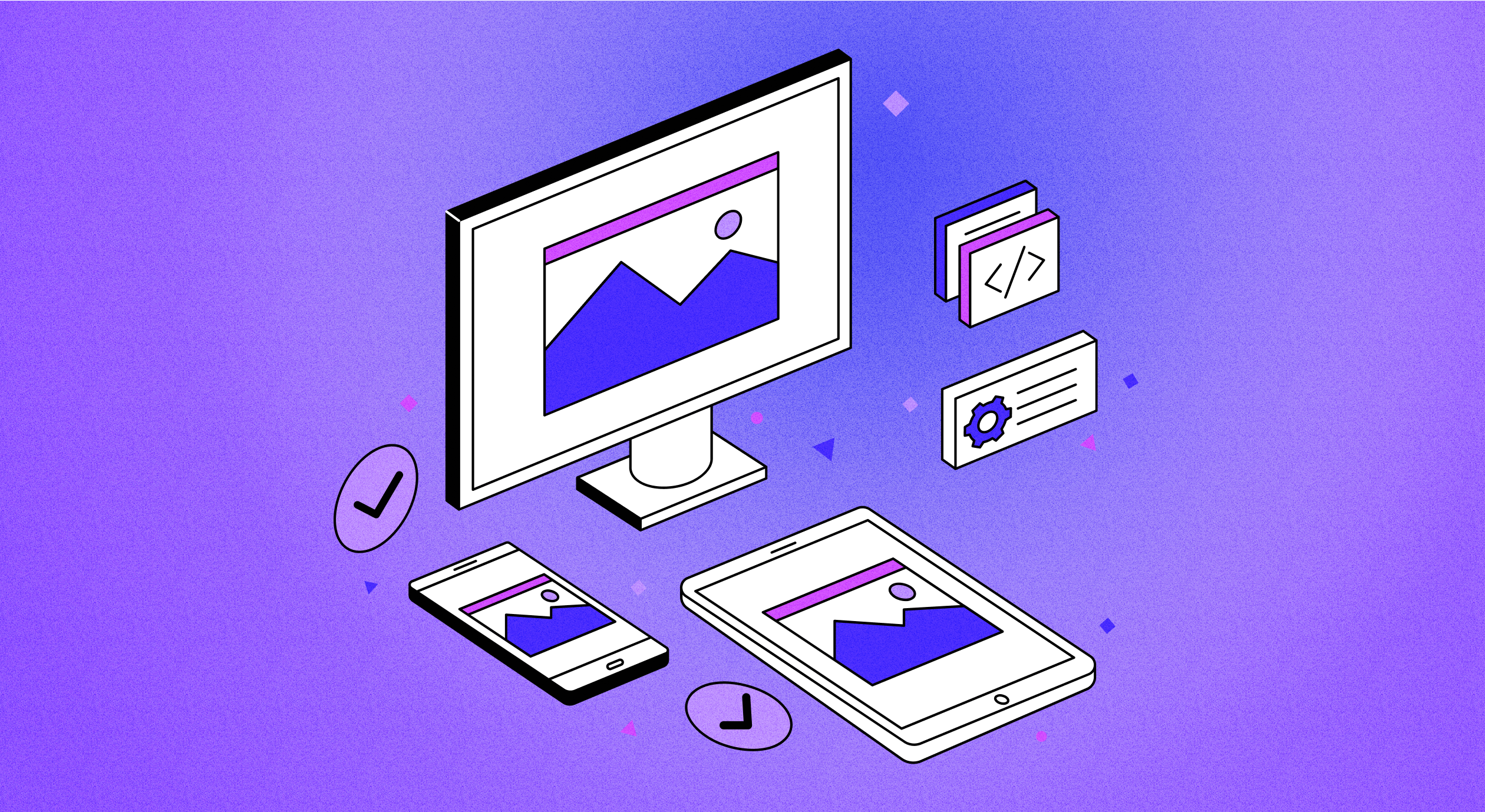
SwiftUI, Apple’s UI toolkit, has dramatically improved life for iOS, iPadOS, and macOS developers. Gone are the days of never-ending code writing or wrestling with Interface Builder to create visually appealing and interactive app screens. With its declarative syntax and robust set of pre-built components, SwiftUI has democratized UI development, making it more accessible and faster than ever before.
Of course, stepping into a new framework can be daunting. That’s why in this article we aim to provide you with a comprehensive guide to getting started with SwiftUI. We’ll cover the basics, explain some key and advanced terms, and offer tips to help you write cleaner, clearer code. Let’s get started.
What is SwiftUI?
Traditionally, iOS apps were primarily built using UIKit, a framework that, while powerful, could be cumbersome and required a deep understanding of its intricacies. SwiftUI has simplified a lot of mundane processes. Now, instead of focusing on how to implement a user interface, developers can concentrate on what they want to achieve, specifying the state and letting SwiftUI take care of the rest. This makes development more intuitive and reduces the time and effort required to bring an app from conception to market. It also ensures that the app design is up to Apple standards, which simplifies the consequent App Store Review process.
SwiftUI is a user interface toolkit introduced by Apple in June 2019 that allows developers to build UIs across all Apple platforms with a single, unified codebase. It is designed to work seamlessly with Swift, Apple’s programming language, and provides a more modern, efficient, and native way to build user interfaces.
Built on a declarative syntax, SwiftUI enables developers to design complex UIs by describing what the interface should look like rather than how it should be built, which is the case in imperative frameworks like UIKit. For example, you can create a button with a title and an action associated with its tap in a very straightforward way, without having to deal with life cycles or delegate methods. All this greatly simplifies the process and unifies the work of several designers by keeping it inside the guidelines.
SwiftUI components
SwiftUI has a robust set of components that serve as the building blocks for creating complex and interactive user interfaces. These components can be broadly categorized into Views, Controls, Data and Events, and Layout Structure, among others. Let’s delve into each to get a better understanding of their roles and functionalities.
Views
In SwiftUI, everything on the screen is a view — text, images, buttons, and even custom-drawn shapes. Views are the basic visual elements that make up your user interface. They can be combined, modified, and nested within each other to build a complete UI.
- Text: For displaying read-only strings.
- Image: For showing images from various sources.
- Shape: For drawing custom shapes like rectangles, circles, etc.
- Spacer: An invisible component that takes up space in layouts.
SwiftUI views are highly customizable and come with a wide array of modifiers like `.padding()
`, `.background()
`, and `.foregroundColor()
` that can be chained together to adjust the view’s appearance and behavior.
Controls
Controls are interactive views that allow users to take actions within the app. SwiftUI provides many built-in controls that handle various kinds of user input.
- Button: For triggering actions.
- Toggle: To switch a setting on or off.
- Slider: To choose a value from a range.
- TextField: To accept textual input from the user.
- Picker: For selecting an item from multiple options.
These controls are designed to automatically adapt to the platform and maintain visual and interactive consistency across devices.
Data and Events
Data management and handling events are crucial in any application. SwiftUI simplifies these aspects with its built-in mechanisms.
- @State: For simple data storage that’s local to a view.
- @Binding: To create a two-way binding between a view and its data.
- @ObservedObject/@Published: For more complex data models that can be shared across views.
- @EnvironmentObject: For sharing data across multiple levels of the view hierarchy.
SwiftUI also integrates with Combine, Apple’s reactive programming framework, for handling events and data flow in a more complex app architecture.
Layout Structure
SwiftUI introduces an intuitive layout system that makes it easy to build complex UIs that adapt to different screen sizes.
HStack
andVStack:
Horizontal and Vertical Stacks for laying out views linearly.ZStack:
For layering views on top of each other.Grid:
For creating more complex two-dimensional layouts.ScrollView:
To display content that can scroll offscreen.
SwiftUI’s layout system is both easy to understand and highly customizable, allowing you to align, space, and size views with simplicity and accuracy.
Others
- Navigation: SwiftUI provides the `
NavigationView
` and `NavigationLink
` components for easy navigation between views. - Animations: Adding animations is incredibly straightforward, often requiring just a single modifier.
- Accessibility: Built-in accessibility features make it easier than ever to create apps that everyone can use.
SwiftUI’s components are designed to be modular, reusable, and easily changeable, allowing developers to build complex UIs without the hours of coding traditionally associated with UI development. Apple-developed components also guarantee that the app will work flawlessly on iOS and will adhere to its design and UX standards. With this comprehensive range of views, controls, and layout structures, SwiftUI quickly became the go-to tool for iOS and macOS developers all over the world.
Building an app with SwiftUI
Xcode 15 set up
- Download and Install: First, download Xcode 15 from the Mac App Store and install it on your Mac.
- Open Xcode: Launch Xcode and select “Create a new Xcode project.”
- Choose Template: Select the “iOS” tab and choose the “App” template.
- Project Details: Fill in the project name, and organization identifier, and make sure to select “SwiftUI” under the “Interface” dropdown.
- Save and Initialize: Choose a location to save your project and click “Create.”
Xcode tour
- Navigator Area: On the left-hand side, you’ll see the navigator where all your project files are listed.
- Editor Area: The central area is where you’ll write your code.
- Utility Area: On the right-hand side, you’ll find the attributes inspector, which allows you to modify various properties of UI elements.
- Toolbar: At the top, you’ll find the toolbar where you can build, run, and debug your app.
- Console: At the bottom, you’ll find the console where logs and debug information appear.
ContentView and preview
When you create a new SwiftUI project in Xcode, you’ll notice a file named `ContentView.swift`. This file contains the default structure for your app’s main view. Here’s what it typically looks like:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, world!")
.padding()
}
}
Here’s what’s inside:
import SwiftUI:
This imports the SwiftUI framework, which provides all the tools and components you need to build user interfaces.struct ContentView: View:
This defines a new SwiftUI view named `ContentView`. In SwiftUI, views are defined as structures that conform to the `View` protocol.var body: some View:
This is a computed property that describes the view’s content and layout. It returns some kind of view, which in this default case is a `Text` view.text("Hello, world!"):
This creates a text label with the specified string..padding():
This is a view modifier that adds padding around the text.
One of the killer features of SwiftUI is the real-time preview. As you write or modify your SwiftUI code, the preview pane immediately reflects those changes. This instant feedback loop allows you to see the impact of their code without having to run the app on a simulator or device.
Not only can you see your UI, but you can also interact with it in the preview pane. This means you can tap buttons, scroll lists, and navigate through your app’s flow right within Xcode. You can also set up multiple previews to display your UI on different device types, orientations, or with different data states. This is incredibly useful for ensuring your UI looks great on all devices and in various conditions.
Creating views
SwiftUI, combined with Xcode’s interface, offers a streamlined approach to creating and designing views. One of the standout features is the drag-and-drop interface, which simplifies the process even for those new to app development. Here’s how it works.
- In your new SwiftUI project, to the right of your code editor, you’ll see the Canvas. This is where the live preview of your SwiftUI code is displayed. If it’s not visible, you can show it by pressing Option + Cmd + Return.
- At the top right of Xcode, there’s a + button. Clicking this will open the Library pane, which contains a collection of SwiftUI views and modifiers. This is your palette for drag-and-drop design.
- Find a view you want to add, like a Button or Image. Click and drag it from the Library pane directly onto the Canvas. As you drag over the Canvas, you’ll see potential drop targets highlighted, showing you where the view can be placed.
- Alternatively, you can drag the view from the Library pane and drop it directly into your code in the editor. Xcode will insert the necessary code for that view at the location you drop it.
- Once you’ve added a view, you can customize its properties using the Attributes Inspector on the right side. This allows you to adjust various attributes without writing code.
- As you drag, drop, and modify views, the Canvas instantly updates, giving you a live preview of your design. You can interact with it just like with a running app.
Combining view modifiers and elements
In SwiftUI, every view is considered immutable: once it’s created, it cannot be changed. However, when you apply a modifier to a view, you’re not actually modifying the view. Instead, you’re creating a new view that wraps the original view with the changes you specified. This approach aligns with SwiftUI’s functional and declarative nature.
Modifiers are chained in the order they are applied, and the order can significantly affect the result. For instance, adding padding before a background color will produce a different effect than adding the color first and then the padding. Play with common view modifiers to see how they influence an element.
Composability in SwiftUI is the ability to create UI components that can be easily combined and nested within one another to build more complex interfaces. In SwiftUI, every view is a composable component, and you can mix and match them as needed. Besides the already familiar HStack, VStack, and ZStack, there’s also Group, List, and ForEach. You can also nest the views within other views and create reusable components that you can recall later anywhere in the app.
As you can see, SwiftUI is a deceptively easy drag-and-drop app editor that drastically simplifies your workflow. Another tool that works just like that is Adapty’s no-code Paywall Builder. With a simple visual editor, you can create several versions of the key converting screen and test them out in your app in a matter of minutes. Try it out now!
10 ideas
to increase
paywall conversion
Get an ebook with insights
and advice from top experts
Best SwiftUI resources
Tutorials from Apple
Coming to the source is always the right first step, and it makes perfect sense to start one’s SwiftUI journey with Apple. Their Developer website is a vast database of knowledge, articles, videos, and coding examples necessary to learn and improve your skills. You can also be sure that the information you are getting is fresh, as the Cupertino company itself is the first one to publish any news or updates on its products.
There are also yearly WWDC (Worldwide Developers Conference) events where you can be among the first to learn about an upcoming feature or a change that will go live only in several months. This, together with helpful developer forums and a strong community, makes the Apple website the go-to source on all things SwiftUI.
Youtube Tutorials
YouTube is the world’s biggest video platform, and one can find information about anything there. That’s why it’s important not to get sucked into the wormhole and keep the research short. YouTube videos work best not for learning the framework from scratch, but rather for resolving a specific problem.
Online Courses
There are many SwiftUI courses, prepared both by professionals and by practicing developers, that introduce the new concepts and help you learn the basics of the frameworks by performing simple tasks or working on a case study. Popular e-education platforms like Udemy and Skillshare have several worthy programs. Be sure to watch the free parts first to see if the course is worth paying for.
SwiftUI Forums
SwiftUI is a popular (if not necessary) framework that attracts and sometimes confuses many developers all over the world. Some of them flock together online on message boards, forums, and Telegram groups. The community is extremely helpful in resolving unusual issues or providing a non-typical way to build a necessary feature.
FAQs
Recommended posts
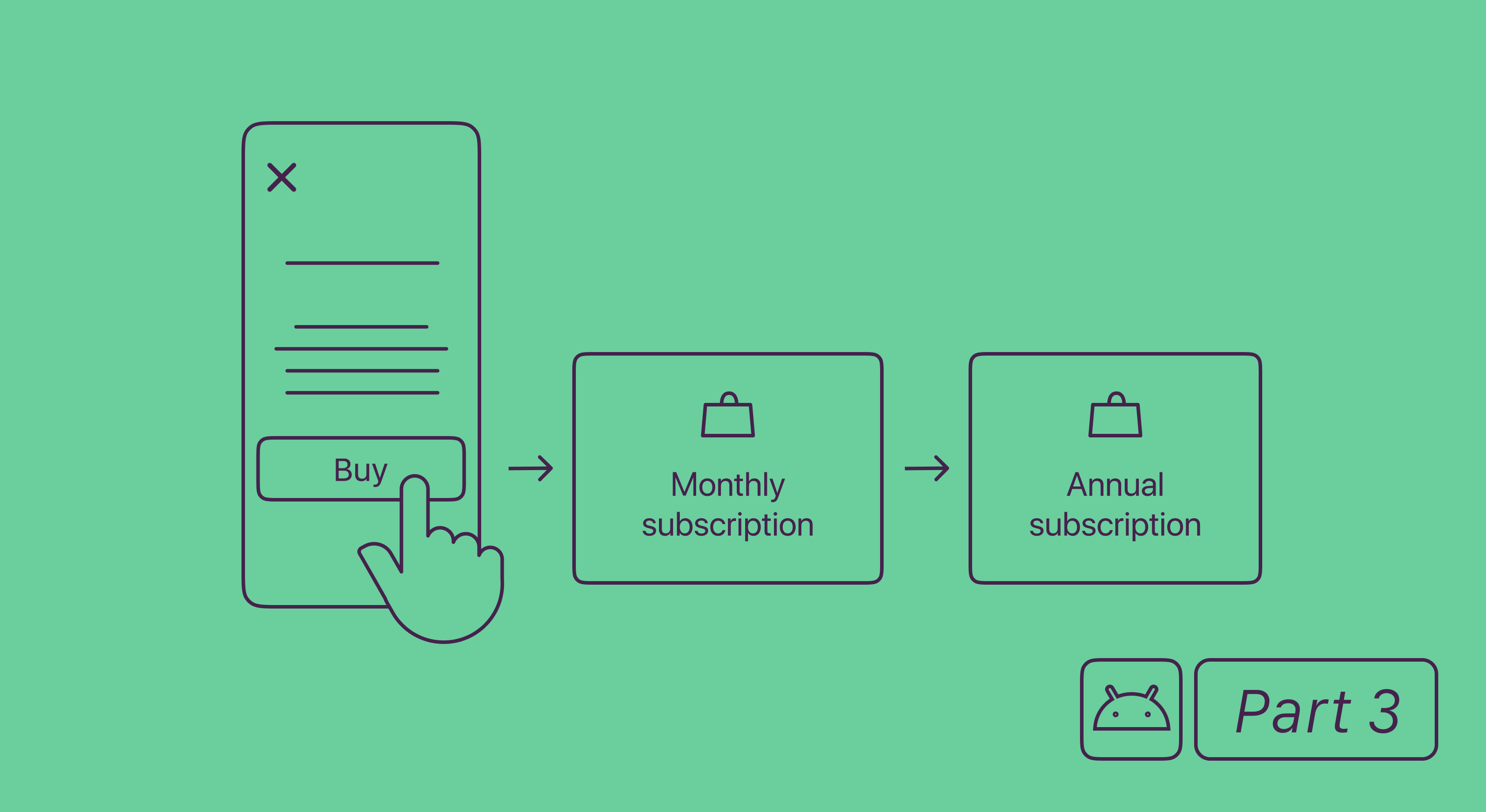
Tutorial
Android
11 min read
August 30, 2021