How to implement in-app purchases using Expo SDK
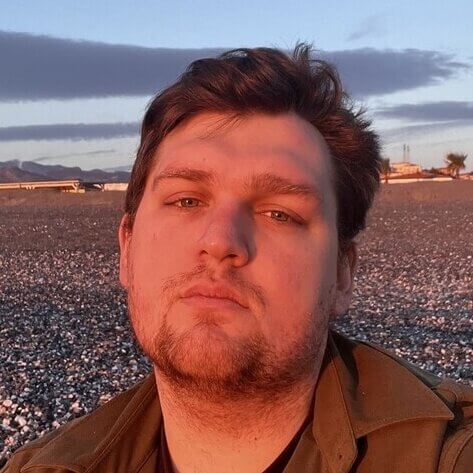
Updated: August 21, 2024
13 min read
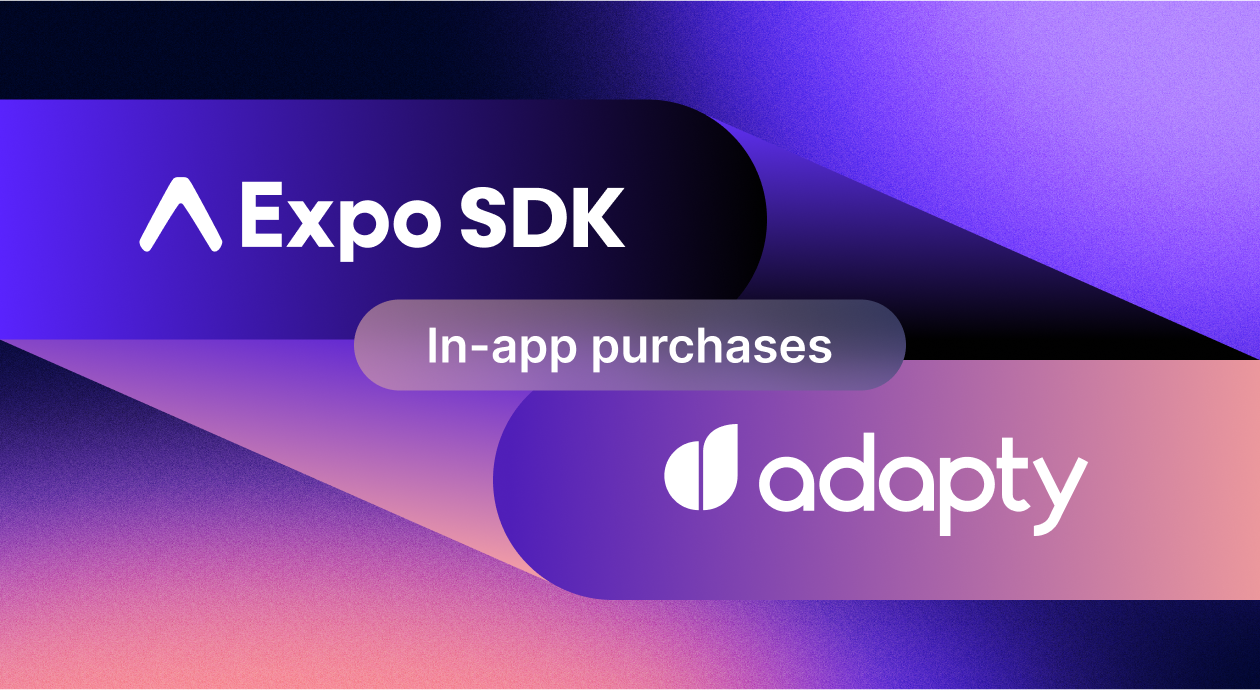
PC programs enjoy a certain degree of simplicity: most games are Windows exclusive, and most design and productivity services come to MacOS first. Mobile app developers, however, have to constantly work and keep updated several versions of their app; not just for the two main mobile OS, but also for different screen sizes and even formats, like foldables and scrollables.
That’s why the industry is in constant search of the one-fixes-all type of solution. One of the options is Expo SDK, which seems to deliver on the promise of universal cross-platform app development, made some years ago by React, Flutter, and the like. In this guide, we briefly present the ecosystem, explain its key features, and show how to make it work with Adapty.
What is Expo SDK?
Expo SDK contains several instruments and shortcuts created around React Native. These tools help teams to develop, ship, and update apps on iOS, Android, and the web from the same JavaScript/TypeScript codebase. The key feature of Expo SDK is its ability to streamline the development process across different platforms, allowing for a single codebase. This allows developers to spend way less time and resources working on and updating their apps
One of Expo’s standout features is its numerous pre-built components and APIs. They simplify the development process by eliminating the need to build certain functionalities from scratch. This shortens the ‘feature-to-user’ timeline and reduces possible bugs. The Expo client app also allows for real-time testing and iteration on physical devices and simulators/emulators, which is crucial for delivering a consistent user experience across all platforms.
Expo SDK automates many tedious and error-prone steps in the build process. Its service can compile apps in the cloud, freeing developers from maintaining native build tooling. Another not-so-obvious benefit of this SDK is the “Over-The-Air” updates feature, which enables developers to publish updates to their apps without going through the app store review process. It provides a time- and nerve-saving advantage in maintaining and improving apps post-release.
How to integrate in-app purchases with Adapty and Expo
Integrating in-app purchases (IAPs) and subscriptions can be a complex process, but tools like Adapty and Expo are specifically designed to help streamline this integration. Here’s what you need to do:
- Install Expo and Adapty SDKs in your project. These SDKs provide the necessary tools and APIs for integrating IAPs. Here’s the instruction for Adapty, and here’s one for Expo.
- Define the in-app products and subscriptions you want to offer within the App Store Connect and Google Play Console. Even if you have them for a long period of time, it’s better to revise and to have only the relevant offers.
- Initialize Adapty in your app with your Adapty API key:
- For a React Native project, call the activate method, passing your public SDK key as an argument:
import { adapty } from 'react-native-adapty';
adapty.activate('PUBLIC_SDK_KEY'); - For an Android project, override the onCreate method. and then call the activate method on Adapty, passing your public SDK key as arguments:
@Override
public void onCreate() {
super.onCreate();
Adapty.activate(getApplicationContext(), "PUBLIC_SDK_KEY"); }
- For a React Native project, call the activate method, passing your public SDK key as an argument:
- Сreate a user-friendly purchase flow within your app with Adapty, showing available products, handling purchases, and managing subscriptions. Defining user-friendly is always a long process, but with our Paywall Builder and Subscription Analytics tools, you can simplify preparing several versions of your offers and make data-powered decisions about their effectiveness.
- Thoroughly test your IAP implementation in sandbox environments provided by Apple and Google. This step is important to ensure that the purchase flow works as expected before going live
- Once tested, deploy your app updates. Continuous monitoring and analysis of in-app purchase and subscription data with Adapty will help you optimize user experience and revenue, and Expo will make it easier to push the necessary updates as soon as possible.
Now let’s look at the key steps in more detail.
Creating projects, installing dependencies, and configuring offers
- First, begin by creating a new project using Expo:
expo init YourProjectName
- Then, navigate to your project directory:
cd YourProjectName
- Install the Expo in-app purchases module which provides the necessary APIs:
expo install expo-in-app-purchases
- Install Adapty SDK to manage and analyze IAPs easily:
npm install @adapty/react-native or yarn add @adapty/react-native
Now you should be able to use both Adapty and Expo correctly, and the next step is introducing the actual offerings. You can set up and configure products by logging into the relevant developer consoles and creating in-app products or subscriptions. For example, in the Google Play Console, navigate to the “Subscriptions” or “In-app products” page, click on the “Create” button, and provide the required product details such as the product ID and name.
Implement ‘react-native-purchases’ in the app
The react-native-purchases library serves as a client for the purchase tracking system, providing a framework around StoreKit and Google Play Billing to simplify IAP implementation in React Native apps. Here’s how you implement it:
- Create a new Bare project:
expo init --template bare-minimum
- Install the required necessities:
npm install --save expo-in-app-purchases
react-native-restart react-native-simple-toast react-native-paper axios - Install Additional Dependencies:
- Install React Navigation prerequisites and React Navigation along with a couple of its navigators — stack and drawer navigation.
- Install
expo-secure-store
for storing sensitive information in the app, and optionally expo-device to determine the user’s device name:expo install react-native-gesture-handler react-native-reanimated react-native-screens react-native-safe-area-context @react-native-community/masked-view
npm install --save @react-navigation/native @react-navigation/stack @react-navigation/drawer
expo install expo-secure-store
expo install expo-device
- Install iOS dependencies: npx pod-install
- Utilize expo-in-app-purchases for implementing in-app purchases within your app.
Integrating react-native-purchases facilitates the management of IAPs by providing a convenient API to perform purchases/restores. It supports features like promo prices to “pay upfront”, which are essential for monetization, better user engagement, and personalized offers. The library supports both Android and iOS, unifying the complexities of each platform’s in-app purchase implementation, ensuring consistent implementation of IAPs across devices, and providing a unified API for simplified integration of IAPs in React Native applications.
React Native app building
Of course, as simplified as Expo makes the multi-platform development process out to be, there are still some specific iOS and Android issues left.
For iOS,
- run sudo gem install cocoapods to install CocoaPods, a dependency manager for Swift and Objective-C Cocoa projects;
- install iOS dependencies using CocoaPods with
cd ios && pod install && cd ..
- ensure you have the correct provisioning profiles set up in Xcode for code signing;
- configure your app icons and launch images through the
Images.xcassets
directory or via Xcode - if your project requires native code, you may need to set up bridging headers for communication between Objective-C/Swift and React Native;
- update the Info.plist file in the iOS folder of your project to include any necessary permissions, configurations, and other metadata; specifically,
- adjust the App Transport Security (ATS) settings in your Info.plist if your app requires network configurations outside the default settings.
For Android,
- generate a signing key using a keystore to sign your app before release;
- install JDK which is required for developing Android applications by running
brew install --cask adoptopenjdk
; - Update the AndroidManifest.xml file in the android/app/src/main directory to include any necessary permissions, configurations, and other metadata.
- optionally, enable ProGuard in your build.gradle file for code obfuscation and optimization;
- Generate a release APK (Android Package) or AAB (Android App Bundle) using the command
cd android && ./gradlew bundleRelease
- if your app requires network configurations outside the default settings, create a network security configuration file and reference it in your AndroidManifest.xml.
Needless to say, all iOS and Android apps are better tested in their native environment, employing XCode and Android Studio, respectively.
React Native app testing
It’s important to test a React Native app on both iOS and Android platforms: there are too many inherent differences between these platforms in terms of user interfaces, behaviors, and system components. Here are some key points you should pay attention to when testing:
- The UI may render differently on iOS and Android due to differences in screen sizes, resolutions, and platform-specific design guidelines. With new device formats, like phones with foldable and rollable screens, keeping the designs uniform is even more difficult. That’s why it’s crucial to double-check that your app always looks correct.
- Each platform has its own set of permissions and security features. Test on both platforms to ensure your app adheres to these, particularly when it comes to transactions and data security surrounding IAPs.
- Test performance is particularly important on Android. There are way more devices running this mobile OS in the world, and they have a wider range of processors, especially worse-performing ones. Usually, apps are limited to certain versions of Android, which in turn are available only on potent enough hardware, but it never hurts to check.
- Ensure that the payment processing systems work flawlessly on both platforms. This includes testing the entire flow of IAPs and subscriptions from item selection and payment processing to the delivery of purchased items. Try out several test purchases and subscriptions, especially features like trial periods and recurring payments, and make sure everything works smoothly, and all payments are registered accordingly.
- Test the receipt validation and purchase restoration processes to ensure that transactions are correctly validated, recorded, and can be restored on both platforms.
- If your app is available in multiple regions, ensure that currency conversions, regional pricing, and localized content are handled correctly. Some currencies have longer names (or prices with more zeros), which can quickly ‘break’ an otherwise perfect paywall.
- Implement and test analytics tools like Adapty to monitor the performance of IAPs on both platforms, which will provide insights into user behavior and transaction success rates.
Testing is a meticulous process but is indispensable for ensuring a seamless, secure, and user-friendly IAP experience across all platforms. By addressing the platform-specific nuances, you significantly enhance the reliability and success of your app’s monetization strategy.
Conclusion
All tools are designed with the idea to simplify a difficult process, but few do it effectively. Expo SDK and Adapty provide a robust framework and insightful analytics respectively, making the process of integrating and managing IAPs significantly more manageable and effective. Both instruments enjoy a high level of user trust and ease of installation, so using them together should be a breeze. But even here, there are some rules to adhere to.
We encourage you to invest some time into reading this article and Adapty and Expo’s documentation. Spending a couple of hours on this today will spend you countless days trying to decipher what went wrong in your app. Together, Expo SDK’s simplification of cross-platform development and Adapty’s adept handling of IAPs form a potent duo that makes sure your apps are easy to keep updated and monetize.