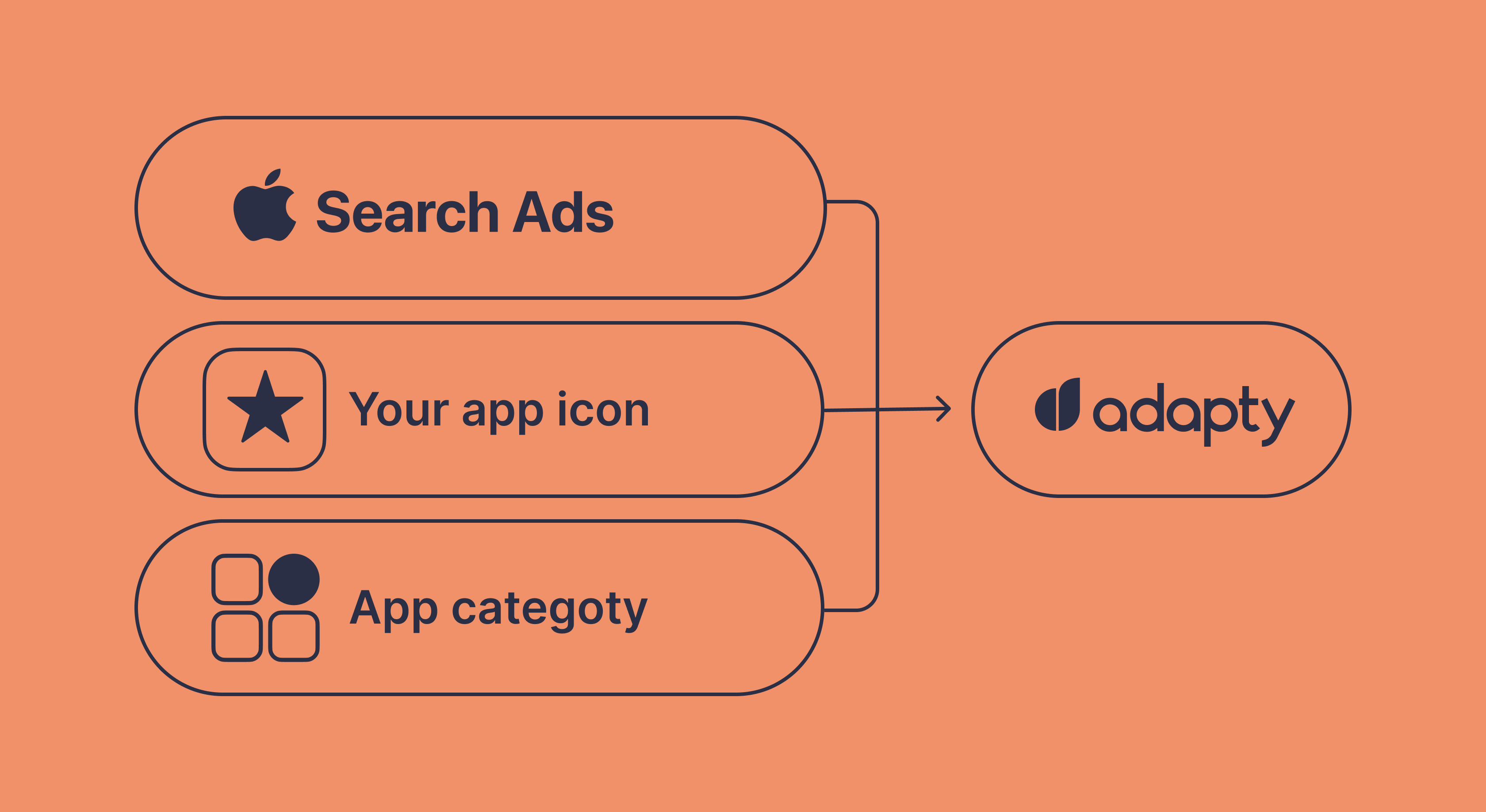
Product-releases
January 13, 2022
Updated: January 16, 2024
20 min read
Welcome to our comprehensive guide on mastering Android UI layout and views. As the backbone of app design, knowing the possibilities and limitations of these tools is essential for developers, and mastering them helps create seamless, intuitive, and visually appealing app interfaces.
We dive deep into the various types of layouts and views, exploring their unique attributes and functionalities. We also provide some examples and best practices for designing and implementing the Views, ensuring that your app looks great and provides an outstanding user experience.
The guide is written from a beginner’s perspective, with some explaining that might seem unnecessary to a more experienced developer. However, it never hurts to go over the basics. Let’s go!
In Android, a View (public class Views
) is the basic building block for creating UI components. Think of it as a small rectangle on the screen that can display content or respond to user interactions. Each View is a fundamental element for creating various types of interactive and display components in an app.
Basic Views include:
Views can also be custom: that is, present a different state of an object. For example, a Button view can change its color or shadow on hover (onHoverEvent) or touch (onTouchEvent). The official Android guide has more examples of Custom Views.
A View Group holds together other Views or even View Groups. It’s essentially a way to combine different Views, allowing for more complex and organized layouts in your app. A View is a basic UI element that is meant to be displayed on the screen, like a button or a text field, a View Group doesn’t display any content of its own but serves as a container to organize and manage other Views.
View Groups provide a structural framework that helps in organizing various Views within a screen. By nesting Views within a View Group, developers can create hierarchical and multi-layered layouts. They are responsible for defining the layout properties of the Views contained within them and often override the basic settings. This includes how these Views are positioned and displayed on the screen.
Layouts in Android serve as the cornerstone for designing and arranging user interfaces. They are essentially like View Groups but are defined by an XML file rather than as a component. They can often include several View Groups and are the map that dictates how different views and elements are organized on the screen. It’s the structural framework that defines the visual structure of a UI.
Mastering the layouts is important because they provide a systematic way to arrange different interface components, ensuring that the app is both aesthetically pleasing and functionally effective. Android devices have a wide range of different screen sizes and resolutions, and layouts help in creating responsive interfaces that adapt seamlessly to various dimensions, with elements like buttons and text fields being logically placed for easy interaction.
Layouts also streamline the development process. By defining a consistent structure for UI elements, they make it easier for developers to implement and modify the app’s interface. A well-structured layout can significantly reduce the complexity of app maintenance, with UI designers and developers being able to change several components instead of entire screens quickly.
You can organize various types of Views and View Groups into different Layouts:
Each layout type serves a unique purpose and understanding when to use which layout is key to creating effective and user-friendly Android applications. Developers choose layouts based on the requirements of the app’s design and the relationships between UI elements.
In Android development, layouts as part of the general UI structure of your app are defined by XML files. Here’s some guidance on their syntax:
android:layout_width="wrap_content" or android:text="Hello World!"
;xmlns:android="http://schemas.android.com/apk/res/android"
.Here are some basic rules about the structure and hierarchy of an XML file:
Mastering XML files takes time, and most developers find their own way to do it. However, there’s some fairly universal advice from us:
<!-- Comment -->
and use them to explain complex parts of your layout;android:id="@+id/name"
: this makes it easier to reference them in your Java or Kotlin code.Here’s a simple example of an XML layout file ерфе shows a LinearLayout containing a TextView and a Button, each with its own attributes. The layout is clear, structured, and easy to understand, highlighting the importance of syntax, structure, and hierarchy:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me" />
</LinearLayout>
You need to load an XML layout into your app to display the UI, and it can be accomplished in 2 ways:
Using setContentView() in an Activity:
This process typically takes place in the onCreate() method. First, you define your XML layout in the res/layout directory. Then, within your Activity, you call setContentView(R.layout.layout_name
), where layout_name is the name of your XML layout file. This method sets the layout for the entire screen of the Activity. Once you’ve called setContentView()
, you can interact with the Views in the XML file using methods like findViewById()
to manipulate them.
Inflating XML layouts as View objects:
This method is a bit different and is mainly used in Fragments, Custom Views, or Adapters. This is where the LayoutInflater class comes into play. It’s used to instantiate layout XML files into their corresponding View objects. For example, in a Fragment, you would use LayoutInflater in the onCreateView()
method to inflate the layout. The process involves passing the layout resource ID, the parent ViewGroup (which is often null in Fragments), and a boolean for whether to attach the inflated layout to the ViewGroup. This method allows for more flexibility as it’s used for creating View hierarchies from XML files for specific parts of the screen, rather than the entire screen.
The choice between using setContentView()
and inflating a layout depends on the context. setContentView()
is specific to Activities and sets the layout for the entire screen, whereas layout inflation offers more flexibility and is used in a variety of contexts, including for individual items in a ListView or for a specific Fragment’s layout. This understanding is crucial for effective UI development in Android, ensuring the appropriate presentation of layouts in different parts of an app.
XML attributes play an important role in defining the properties and behavior of UI elements. Among these, layout_width and layout_height are two of the most commonly used attributes. They are essential for determining the size of Views and ViewGroups. Here are some common XML attributes and what they can be used for.
Attribute | Purpose |
---|---|
layout_width | Specifies the width of a View or ViewGroup. Common values include match_parent, wrap_content, and specific dimensions. |
layout_height | Specifies the height of a View or ViewGroup. Like layout_width, it can be match_parent, wrap_content, or a specific size. |
layout_gravity | Used in certain ViewGroups like LinearLayout or FrameLayout to specify the position of a View within the ViewGroup. |
padding or margin | padding defines the space inside the View boundaries, while margin defines the space outside, around the View. |
id | Assigns a unique identifier to a View, which is crucial for referencing the View in your Java or Kotlin code. |
src | Used in ImageView to define the source image. |
text | Sets the text displayed by a TextView or a Button. |
orientation | Defines the direction (vertical or horizontal) in ViewGroups like LinearLayout. |
gravity | Specifies the alignment of a View’s content, such as text in a TextView or Button. |
These attributes are integral to the design and functionality of your app interface. layout_width
and layout_height
are mandatory for every View and ViewGroup, as they dictate their size. Attributes like layout_gravity
, padding, and margin further refine the positioning and spacing, greatly helping to create a polished look on any device. The id
attribute is essential for programmatically manipulating Views, such as setting listeners or altering their properties at runtime. Meanwhile, attributes like src
, text
, and orientation
are more specific to the type of View and define their primary characteristics.
Understanding and effectively using these XML attributes is key to creating functional, well-designed Android apps. They allow developers to create interfaces that are not only visually appealing but also user-friendly and responsive to different device sizes and orientations.
2024 subscription benchmarks and insights
Get your free copy of our latest subscription report to stay ahead in 2024.
Coming up with efficient, responsive, and accessible UI layouts for Android apps involves a blend of best practices and easy-to-pick-up tips to ensure an appealing, functional, and inclusive user experience. Here’s how you can achieve this:
match_parent
, wrap_content
, and weight
in LinearLayout or constraints in ConstraintLayout. Always remember to test your application on a variety of devices and screen sizes to ensure scalability and prevent any failures. Sometimes, combining different layouts may yield the best results across various screens.Incorporating these strategies enhances user experience, making your app more accessible and usable by a diverse audience. Regular testing and feedback, especially from users with disabilities, are invaluable for continuous improvement in the accessibility and usability of your app.
Recommended posts
Product-releases
January 13, 2022